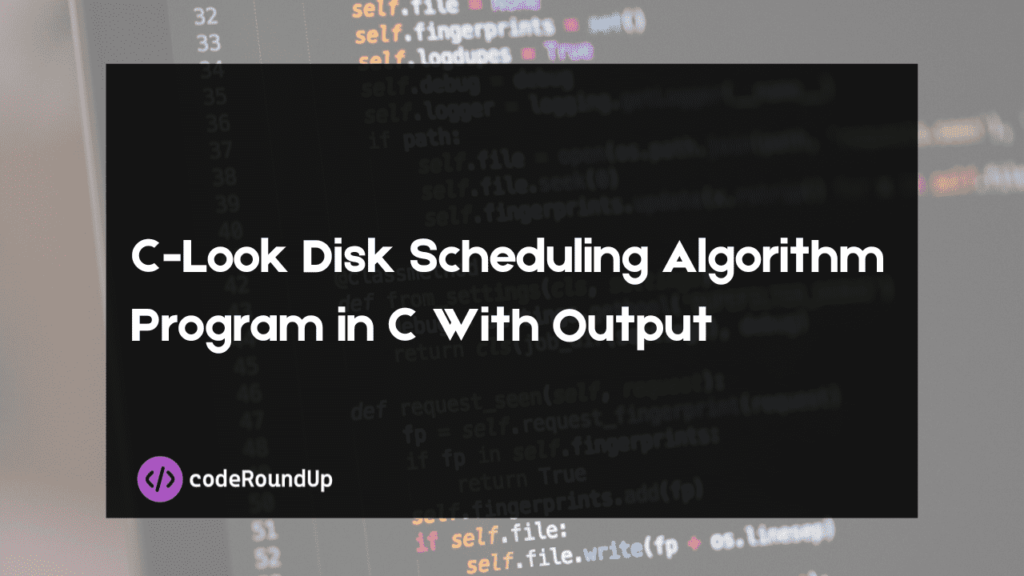
Discover efficient disk management with our “C-Look Disk Scheduling Algorithm Program in C.” Disk scheduling algorithms play a crucial role in optimizing data access on computer systems. Among various algorithms, the C-Look Disk Scheduling Algorithm stands out for its efficiency and practicality. In this article, we’ll dive into the complexity of C-Look, we will explore how it works, help you through implementing it in C language, and discuss its advantages and disadvantages.
Understanding C-Look Disk Scheduling Algorithm
In the realm of disk scheduling algorithms, C-Look is renowned for its simplicity and effectiveness. Unlike other algorithms, C-Look exhibits a unique circular scanning mechanism, prioritizing speed and efficiency. Let’s compare it with other disk scheduling algorithms to grasp its significance.
How C-Look Works
C-Look operates on the principles of the Scan and Look algorithms, optimizing disk access by minimizing seek time. Its circular scanning mechanism ensures quick and efficient data retrieval. Understanding the specifics of C-Look is essential for harnessing its advantages over other scheduling algorithms.
C-Look Disk Scheduling Algorithm:
The C-Look algorithm is a disk scheduling algorithm that optimizes the movement of the disk arm to reduce seek time. It is an improvement over the traditional Look algorithm. C-Look is designed to be more efficient and predictable in servicing I/O requests.
Initialization:
The disk arm starts at one end of the disk and moves towards the other end in a specific direction.
Request Queue:
Incoming I/O requests are sorted based on their track numbers in the request queue.
Scanning Direction:
Unlike the Look algorithm, C-Look does not change its direction when reaching the end of the disk. Instead, it immediately moves back to the starting point without servicing requests on the return journey.
Servicing Requests:
The algorithm services requests in the direction of the disk arm movement until all requests in that direction are satisfied.
Returning to Starting Point:
Once all requests in the current direction are serviced, the disk arm quickly moves back to the starting point without servicing requests on the return journey.
Repeating the Process:
The process continues, with the disk arm oscillating back and forth, servicing requests in the direction it is moving.
C-Look Disk Scheduling Algorithm Program in C With Output
Now, let’s get hands-on and implement C-Look in C programming. Follow this step-by-step guide and explore code snippets to comprehend the process. Coding enthusiasts will find this section particularly insightful, providing a practical approach to mastering C-Look Disk Scheduling Algorithm Program in C.
Want more deep insights on C-Look click here.
#include <stdio.h>
#include <stdlib.h>
// Function to sort the array in ascending order
void sortDiskRequests(int diskRequests[], int size) {
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (diskRequests[j] > diskRequests[j + 1]) {
// Swap elements if they are in the wrong order
int temp = diskRequests[j];
diskRequests[j] = diskRequests[j + 1];
diskRequests[j + 1] = temp;
}
}
}
}
// C-Look Disk Scheduling Algorithm
void cLookDiskScheduling(int diskRequests[], int initialHead, int requestCount, int totalDiskSize, int headMovementDirection) {
// Sort the array in ascending order
sortDiskRequests(diskRequests, requestCount);
int totalHeadMovement = 0;
int distance, currentTrack;
// Find the index where the initial head position falls in the sorted sequence
int index;
for (int i = 0; i < requestCount; i++) {
if (initialHead < diskRequests[i]) {
index = i;
break;
}
}
// Calculate total head movement based on the C-Look algorithm
if (headMovementDirection == 1) {
for (int i = index; i < requestCount; i++) {
currentTrack = diskRequests[i];
distance = abs(currentTrack - initialHead);
totalHeadMovement += distance;
initialHead = currentTrack;
}
for (int i = 0; i < index; i++) {
currentTrack = diskRequests[i];
distance = abs(currentTrack - initialHead);
totalHeadMovement += distance;
initialHead = currentTrack;
}
} else {
for (int i = index - 1; i >= 0; i--) {
currentTrack = diskRequests[i];
distance = abs(currentTrack - initialHead);
totalHeadMovement += distance;
initialHead = currentTrack;
}
for (int i = requestCount - 1; i >= index; i--) {
currentTrack = diskRequests[i];
distance = abs(currentTrack - initialHead);
totalHeadMovement += distance;
initialHead = currentTrack;
}
}
// Print the total head movement
printf("Total head movement is %d\n", totalHeadMovement);
}
int main() {
int diskRequests[100], initialHead, requestCount, totalDiskSize, headMovementDirection;
// Get user input for the number of requests
printf("Enter the number of Requests\n");
scanf("%d", &requestCount);
// Get user input for the request sequence
printf("Enter the Requests sequence\n");
for (int i = 0; i < requestCount; i++)
scanf("%d", &diskRequests[i]);
// Get user input for initial head position and total disk size
printf("Enter initial head position\n");
scanf("%d", &initialHead);
printf("Enter total disk size\n");
scanf("%d", &totalDiskSize);
// Get user input for the head movement direction (1 for high, 0 for low)
printf("Enter the head movement direction for high (1) and for low (0)\n");
scanf("%d", &headMovementDirection);
// Apply C-Look Disk Scheduling
cLookDiskScheduling(diskRequests, initialHead, requestCount, totalDiskSize, headMovementDirection);
return 0;
}
Output:
Enter the number of Requests
5
Enter the Requests sequence
23
45
67
89
25
Enter initial head position
20
Enter total disk size
10
Enter the head movement direction for high (1) and for low (0)
1
Total head movement is 69
Advantages of C-Look Disk Scheduling Algorithm
Reduced Seek Time:
C-Look’s circular scanning mechanism minimizes seek time by focusing on a specific range of tracks. This results in faster data retrieval compared to some other disk scheduling algorithms.
Improved Throughput:
The algorithm’s efficiency in handling read and write requests enhances overall system throughput. This makes C-Look a preferred choice in scenarios where high data throughput is crucial.
Simple Implementation:
C-Look is relatively simple to implement compared to more complex algorithms. Its straightforward approach makes it accessible for developers and contributes to quicker development cycles.
Predictable Performance:
C-Look’s deterministic nature ensures predictable performance, making it easier for system administrators and developers to anticipate and manage disk access times.
Suitability for Certain Workloads:
In scenarios where the workload involves frequent access to a specific range of tracks, C-Look excels. Its circular scanning pattern makes it well-suited for such situations.
Disadvantages of C-Look Disk Scheduling Algorithm
Unfairness in Track Servicing:
C-Look may exhibit unfairness in servicing requests, particularly if there’s a concentration of requests in the specific range that the algorithm is currently scanning. Requests outside this range may experience delays.
Limited Adaptability:
While effective in certain scenarios, C-Look may not adapt well to varying workloads or disk access patterns. This lack of adaptability can be a drawback in dynamic computing environments.
Potential for Starvation:
In situations where there’s a continuous influx of requests in the specific range being scanned, tracks outside this range may experience starvation, leading to delayed service.
Not Ideal for Every Scenario:
C-Look’s efficiency is context-dependent. It may not be the best choice for scenarios with unpredictable or highly dynamic disk access patterns, where other algorithms like SCAN might perform better.
Limited Optimization for Write Requests:
While C-Look is effective for read-intensive workloads, it may not be optimized for scenarios where there’s a significant volume of write requests. Other algorithms may better handle such situations.
Conclusion
To sum up, the disk scheduling algorithm C-Look provides a special method for maximizing data access on storage devices. We’ve covered its basics, implementation in C, examining its benefits and drawbacks are essential actions for developers looking to improve system efficiency.
Want to learn more.!? From syntax to algorithms, our C tutorial covers it all in a compact format.
FAQ
What is the C-look algorithm in disk scheduling?
C-Look is a disk scheduling algorithm that scans the disk in a one-way direction, servicing requests until the end, then swiftly returning to the beginning without considering the unvisited requests.
What is the Cscan disk scheduling algorithm?
C-Scan is a disk scheduling method that acts like C-Look but with the added feature of moving only in one direction and restarting from the beginning when reaching the end, preventing unnecessary backward movement.
What are the 6 scheduling algorithms?
The six common disk scheduling algorithms are First-Come-First-Serve (FCFS), Shortest Seek Time First (SSTF), SCAN, C-Scan, C-Look, and Elevator (SCAN with a look-ahead). Each algorithm employs unique strategies to enhance disk I/O performance in specific scenarios.
What are the disadvantages of C-look disk scheduling?
The main disadvantage of C-Look is its potential for increased waiting time for certain requests, especially those located far from the disk arm’s current position. It may not provide optimal performance in scenarios with varied request patterns.
What are the 6 scheduling algorithms?
The six common disk scheduling algorithms are First-Come-First-Serve (FCFS), Shortest Seek Time First (SSTF), SCAN, C-Scan, C-Look, and Elevator (SCAN with a look-ahead). Each algorithm employs unique strategies to enhance disk I/O performance in specific scenarios.