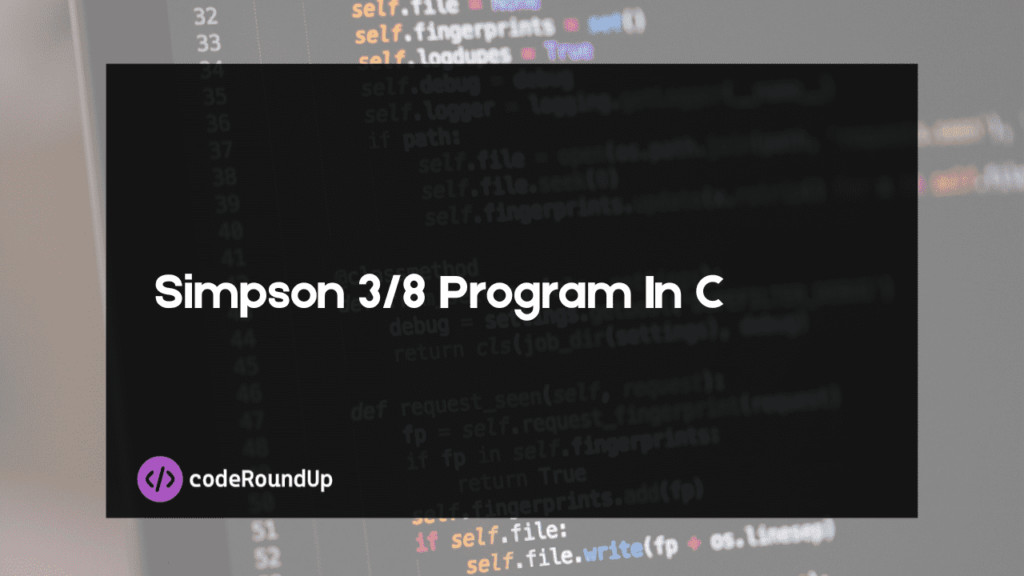
The Simpson 3/8 Rule in C is a reliable method for numerical analysis and calculating definite integrals. The article will examine the features and benefits of the Simpson 3/8 program in C, as well as its implementation, importance, and practical uses.
Understanding Simpson’s 3/8 Rule
The accuracy of the numerical integration technique Simpson’s Rule is well known. The factor used in the formula is denoted by the 3/8 in Simpson’s 3/8 Rule. Let’s study the mathematical formula behind this rule and break it down to properly understand it.
Implementing Simpson 3/8 Rule in C
Now, let’s roll up our sleeves and dive into the practical aspect. Setting up the C programming environment is the first step, followed by a detailed guide on coding Simpson 3/8 Rule. Common challenges faced during implementation will also be addressed with effective solutions.
Formula
∫ab f(x) dx = 3h/8 [(y0 + yn) + 3(y1 + y2 + y4 + y5 + …. + yn-1) + 2(y3 + y6 + y9 + ….. + yn-3)]
Advantages of Using Simpson 3/8 Rule Method
Why choose Simpson’s 3/8 Rule over other numerical methods? We’ll explore the accuracy it brings in approximating definite integrals, its efficiency, and delve into real-world applications where this method shines.
Limitations and Considerations
Every method has its limitations. We’ll discuss scenarios where Simpson’s 3/8 Rule might not be the optimal choice and provide insights on addressing potential errors and inaccuracies.
Simpson 3/8 Program in C
#include<stdio.h>
#include<math.h>
double f(double x)
{
return x*x;
}
main()
{
int n,i;
double a,b,h,x,sum=0,integral;
printf("\nEnter the no. of sub-intervals(MULTIPLE OF 3): ");
scanf("%d",&n);
printf("\nEnter the initial limit: ");
scanf("%lf",&a);
printf("\nEnter the final limit: ");
scanf("%lf",&b);
h=fabs(b-a)/n;
for(i=1;i<n;i++)
{
x=a+i*h;
if(i%3==0)
{
sum=sum+2*f(x);
}
else
{
sum=sum+3*f(x);
}
}
integral=(3*h/8)*(f(a)+f(b)+sum);
printf("\nThe integral is: %lf\n",integral);
}
Output
Enter the no. of sub-intervals(MULTIPLE OF 3): 99
Enter the initial limit: 0
Enter the final limit: 3
The integral is: 9.000000
Want to read more on Simpson’s rule? Click here.
Comparing Simpson 3/8 Rule with Other Numerical Methods
To give readers a comprehensive understanding, a comparative analysis with Simpson’s 1/3 Rule and trapezoidal rule will be presented. This section will aid in choosing the right method for specific scenarios.
Tips for Efficient Implementation
Optimizing the code for better performance is crucial. We’ll provide tips on achieving efficiency, understanding the importance of precision, and incorporating user-friendly features in the program.
Real-world Applications
Where does Simpson 3/8 Rule find practical use? We’ll explore various fields, share success stories, and discuss the method’s future prospects and advancements.
Common Mistakes to Avoid
No program is without its pitfalls. We’ll help readers identify and rectify common programming errors, ensure proper input and output handling, and provide debugging techniques specific to Simpson 3/8 Rule programs.
User Experiences and Testimonials
Learn from those who have walked the path. This section will present insights from programmers who have used Simpson 3/8 Rule, detailing challenges faced, lessons learned, and how the method contributed to successful projects.
Conclusion
In conclusion, Simpson’s 3/8 Rule in C is a valuable tool in numerical analysis. From its theoretical underpinnings to real-world applications, we’ve explored the ins and outs. Encouraging readers to delve into the method’s implementation, this article aims to empower programmers with the knowledge to leverage Simpson 3/8 Rule effectively.
Interested in learning C programming? Click here!
FAQ
What is Simpson’s 3/8 in C program?
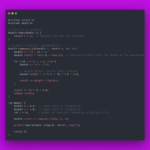
Simpson’s 3/8 rule is implemented in a C program to numerically integrate a given function. It divides the interval into subintervals, calculates function values, and applies the 3/8 rule formula for more accurate approximation.
#include <stdio.h>
#include <math.h>
// Function to integrate
double func(double x) {
return x * x; // Replace with your own function
}
// Simpson’s 3/8 Rule implementation
double simpsons_3_8(double a, double b, int n) {
double h = (b – a) / n;
double result = func(a) + func(b); // Initialize result with the values at the boundaries
for (int i = 1; i < n; i++) { double x = a + i * h; // Weight factor: 3/8 for every interval double weight = (i % 3 == 0) ? 2.0 : 3.0; result += weight * func(x); } result *= (3 * h) / 8.0; return result;
}
int main() {
double a = 0.0; // Lower limit of integration
double b = 1.0; // Upper limit of integration
int n = 6; // Number of intervals (should be a multiple of 3)
double result = simpsons_3_8(a, b, n); printf(“Approximate integral: %lf\n”, result); return 0;
}
What is the Simpson’s 3/8 rule condition?
The Simpson’s 3/8 rule is applicable when the number of intervals (n) is a multiple of 3, ensuring that each interval can be divided into three subintervals.
What is the degree of precision of Simpson’s 3/8 rule?
Simpson’s 3/8 rule has a degree of precision of 3, meaning it provides an exact result for polynomials of degree up to 3.
What should be the number of intervals in Simpson’s 3/8 rule?
The number of intervals (n) in Simpson’s 3/8 rule should be a multiple of 3 to satisfy the condition of the method.
What is the difference between Simpson’s rule and Simpson’s 3/8 rule?
Simpson’s rule approximates the integral using quadratic polynomials, while Simpson’s 3/8 rule uses cubic polynomials. The 3/8 rule is more accurate but requires the number of intervals to be a multiple of 3.
What is the Simpson’s first rule?
Simpson’s first rule is a simpler version that uses quadratic polynomials to approximate the integral. It is a special case of Simpson’s rule when the number of intervals is even.
What is Simpson’s rule example?
For example, in the interval [a, b], if f(x) = x^2, applying Simpson’s rule would involve calculating the area under the curve using quadratic approximations for accurate integration.